It's an exciting time for React as there are now more commits from open source contributors than from Facebook engineers! Keep up the good work :)
Atom moves to React #
Atom, GitHub's code editor, is now using React to build the editing experience. They made the move in order to improve performance. By default, React helped them eliminate unnecessary reflows, enabling them to focus on architecting the rendering pipeline in order to minimize repaints by using hardware acceleration. This is a testament to the fact that React's architecture is perfect for high performant applications.
Why Does React Scale? #
At the last JSConf.us, Vjeux talked about the design decisions made in the API that allows it to scale to a large number of developers. If you don't have 20 minutes, take a look at the annotated slides.
Live Editing #
One of the best features of React is that it provides the foundations to implement concepts that were otherwise extremely difficult, like server-side rendering, undo-redo, rendering to non-DOM environments like canvas... Dan Abramov got hot code reloading working with webpack in order to live edit a React project!
ReactIntl Mixin by Yahoo #
There are a couple of React-related projects that recently appeared on Yahoo's GitHub, the first one being an internationalization mixin. It's great to see them getting excited about React and contributing back to the community.
var MyComponent = React.createClass({
mixins: [ReactIntlMixin],
render: function() {
return (
<div>
<p>{this.intlDate(1390518044403, { hour: 'numeric', minute: 'numeric' })}</p>
<p>{this.intlNumber(400, { style: 'percent' })}</p>
</div>
);
}
});
React.renderComponent(
<MyComponent locales={['fr-FR']} />,
document.getElementById('example')
);
Thinking and Learning React #
Josephine Hall, working at Icelab, used React to write a mobile-focused application. She wrote a blog post “Thinking and Learning React.js” to share her experience with elements they had to use. You'll learn about routing, event dispatch, touchable components, and basic animations.
London React Meetup #
If you missed the last London React Meetup, the video is available, with lots of great content.
- What's new in React 0.11 and how to improve performance by guaranteeing immutability
- State handling in React with Morearty.JS
- React on Rails - How to use React with Ruby on Rails to build isomorphic apps
- Building an isomorphic, real-time to-do list with moped and node.js
In related news, the next React SF Meetup will be from Prezi: “Immediate Mode on the Web: How We Implemented the Prezi Viewer in JavaScript”. While not in React, their tech is really awesome and shares a lot of React's design principles and perf optimizations.
Using React and KendoUI Together #
One of the strengths of React is that it plays nicely with other libraries. Jim Cowart proved it by writing a tutorial that explains how to write React component adapters for KendoUI.
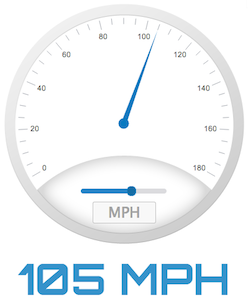
Acorn JSX #
Ingvar Stepanyan extended the Acorn JavaScript parser to support JSX. The result is a JSX parser that's 1.5–2.0x faster than the official JSX implementation. It is an experiment and is not meant to be used for serious things, but it's always a good thing to get competition on performance!
ReactScriptLoader #
Yariv Sadan created ReactScriptLoader to make it easier to write components that require an external script.
var Foo = React.createClass({
mixins: [ReactScriptLoaderMixin],
getScriptURL: function() {
return 'http://d3js.org/d3.v3.min.js';
},
getInitialState: function() {
return { scriptLoading: true, scriptLoadError: false };
},
onScriptLoaded: function() {
this.setState({scriptLoading: false});
},
onScriptError: function() {
this.setState({scriptLoading: false, scriptLoadError: true});
},
render: function() {
var message =
this.state.scriptLoading ? 'Loading script...' :
this.state.scriptLoadError ? 'Loading failed' :
'Loading succeeded';
return <span>{message}</span>;
}
});
Random Tweet #
“@apphacker: I take back the mean things I said about @reactjs I actually like it.” Summarizing the life of ReactJS in a single tweet.
— Jordan (@jordwalke) July 20, 2014