This round-up is a special edition on Flux. If you expect to see diagrams showing arrows that all point in the same direction, you won't be disappointed!
React And Flux at ForwardJS #
Facebook engineers Jing Chen and Bill Fisher gave a talk about Flux and React at ForwardJS, and how using an application architecture with a unidirectional data flow helped solve recurring bugs.
Yahoo #
Yahoo is converting Yahoo Mail to React and Flux and in the process, they open sourced several components. This will help you get an isomorphic application up and running.
Reflux #
Mikael Brassman wrote Reflux, a library that implements Flux concepts. Note that it diverges significantly from the way we use Flux at Facebook. He explains the reasons why in a blog post.
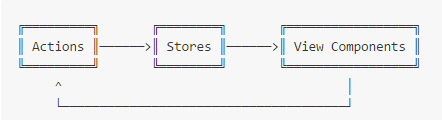
React and Flux Interview #
Ian Obermiller, engineer at Facebook, made a lengthy interview on the experience of using React and Flux in order to build probably the biggest React application ever written so far.
I’ve actually said this many times to my team too, I love React. It’s really great for making these complex applications. One thing that really surprised me with it is that React combined with a sane module system like CommonJS, and making sure that you actually modulize your stuff properly has scaled really well to a team of almost 10 developers working on hundreds of files and tens of thousands of lines of code.
Really, a fairly large code base... stuff just works. You don’t have to worry about mutating, and the state of the DOM just really makes stuff easy. Just conceptually it’s easier just to think about here’s what I have, here’s my data, here’s how it renders, I don’t care about anything else. For most cases that is really simplifying and makes it really fast to do stuff.
Adobe's Brackets Project Tree #
Kevin Dangoor is converting the project tree of Adobe's Bracket text editor to React and Flux. He wrote about his experience using Flux.
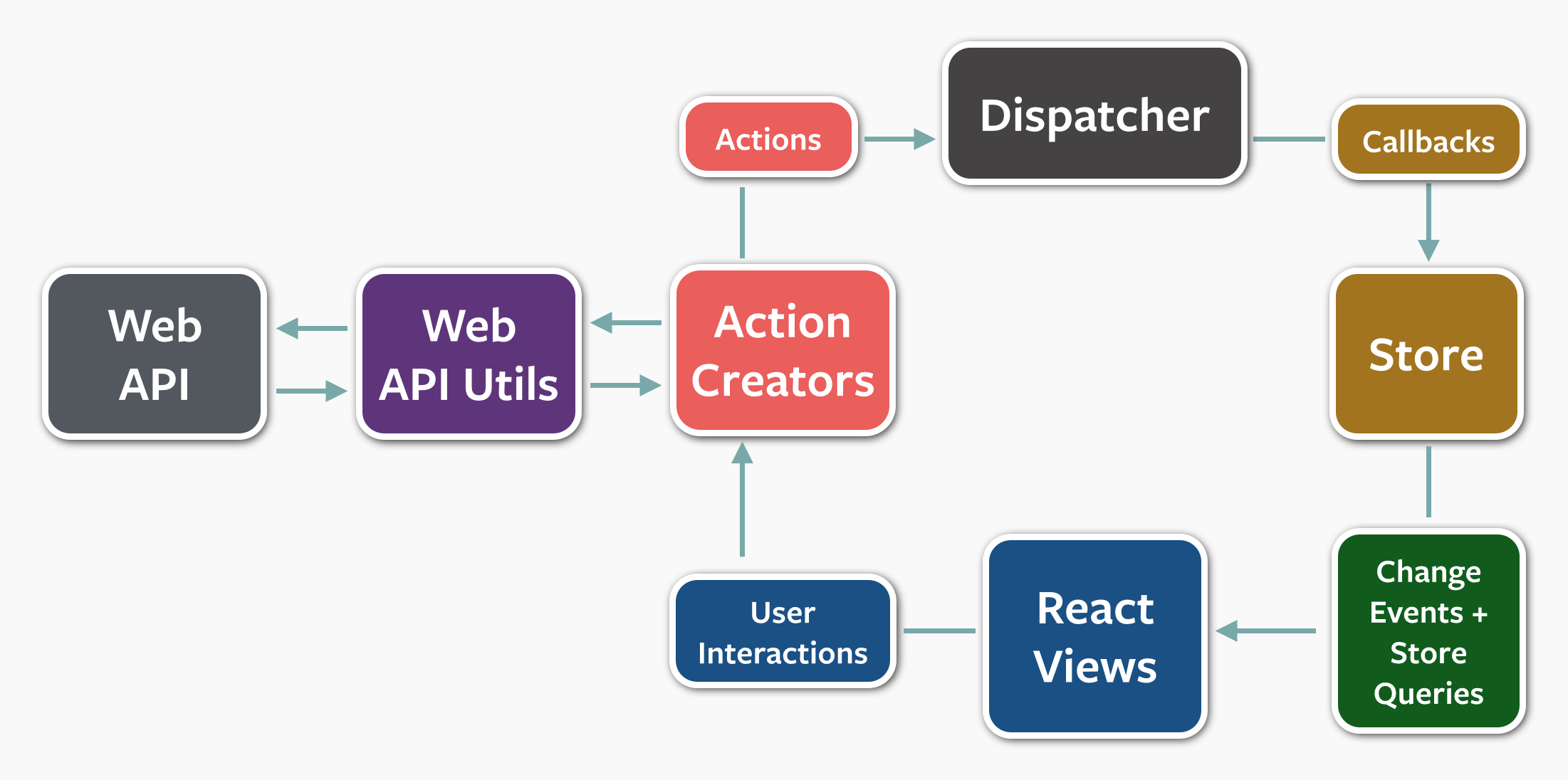
Async Requests with Flux Revisited #
Reto Schläpfer came back to a Flux project he hasn't worked on for a month and saw many ways to improve the way he implemented Flux. He summarized his learnings in a blog post.
The smarter way is to call the Web Api directly from an Action Creator and then make the Api dispatch an event with the request result as a payload. The Store(s) can choose to listen on those request actions and change their state accordingly.
Before I show some updated code snippets, let me explain why this is superior:
There should be only one channel for all state changes: The Dispatcher. This makes debugging easy because it just requires a single console.log in the dispatcher to observe every single state change trigger.
Asynchronously executed callbacks should not leak into Stores. The consequences of it are just to hard to fully foresee. This leads to elusive bugs. Stores should only execute synchronous code. Otherwise they are too hard to understand.
Avoiding actions firing other actions makes your app simple. We use the newest Dispatcher implementation from Facebook that does not allow a new dispatch while dispatching. It forces you to do things right.
Undo-Redo with Immutable Data Structures #
Ameya Karve explained how to use Mori, a library that provides immutable data structures, in order to implement undo-redo. This usually very challenging feature only takes a few lines of code with Flux!
undo: function() {
this.redoStates = Mori.conj(this.redoStates, Mori.first(this.undoStates));
this.undoStates = Mori.drop(1, this.undoStates);
this.todosState = Mori.first(this.undoStates);
this.canUndo = Mori.count(this.undoStates) > 1;
this.canRedo = true;
if (Mori.count(this.undoStates) > 1) {
this.todos = JSON.parse(this.todosState);
} else {
this.todos = [];
}
this.emit('change');
},
Flux in practice #
Gary Chambers wrote a guide to get started with Flux. This is a very practical introduction to Flux.
So, what does it actually mean to write an application in the Flux way? At that moment of inspiration, when faced with an empty text editor, how should you begin? This post follows the process of building a Flux-compliant application from scratch.
Components, React and Flux #
Dan Abramov working at Stampsy made a talk about React and Flux. It's a very good overview of the concepts at play.
React and Flux #
Christian Alfoni wrote an article where he compares Backbone, Angular and Flux on a simple example that's representative of a real project he worked on.
Wow, that was a bit more code! Well, try to think of it like this. In the above examples, if we were to do any changes to the application we would probably have to move things around. In the FLUX example we have considered that from the start.
Any changes to the application is adding, not moving things around. If you need a new store, just add it and make components dependant of it. If you need more views, create a component and use it inside any other component without affecting their current "parent controller or models".
Flux: Step by Step approach #
Nicola Paolucci from Atlassian wrote a great guide to help your getting understand Flux step by step.
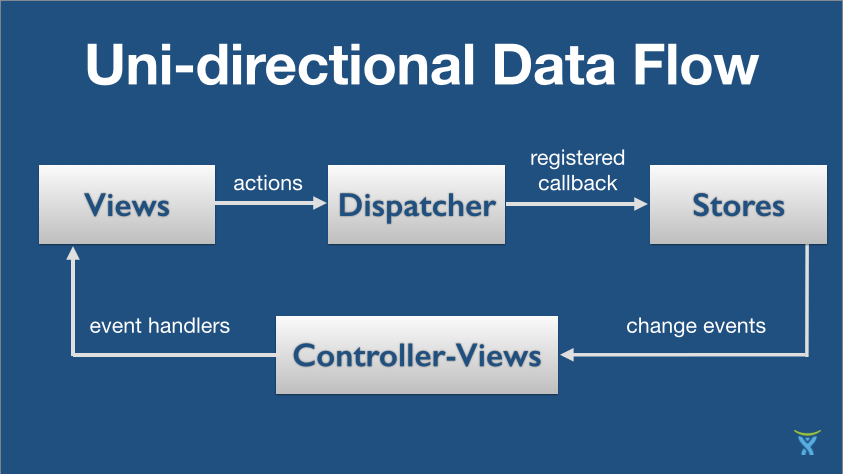
DeLorean: Back to the future! #
DeLorean is a tiny Flux pattern implementation developed by Fatih Kadir Akin.
- Unidirectional data flow, it makes your app logic simpler than MVC
- Automatically listens to data changes and keeps your data updated
- Makes data more consistent across your whole application
- It's framework agnostic, completely. There's no view framework dependency
- Very small, just 4K gzipped
- Built-in React.js integration, easy to use with Flight.js and Ractive.js and probably all others
- Improve your UI/data consistency using rollbacks
Facebook's iOS Infrastructure #
Last but not least, Flux and React ideas are not limited to JavaScript inside of the browser. The iOS team at Facebook re-implemented Newsfeed using very similar patterns.
Random Tweet #
If you build your app with flux, you can swap out React for a canvas or svg view layer and keep 85% of your code. (or the thing after React)
— Ryan Florence (@ryanflorence) September 3, 2014