This has been an exciting summer as four big companies: Yahoo, Mozilla, Airbnb and Reddit announced that they were using React!
|
|
|
|
React's Architecture #
Vjeux, from the React team, gave a talk at OSCON on the history of React and the various optimizations strategies that are implemented. You can also check out the annotated slides or Chris Dawson's notes titled JavaScript’s History and How it Led To React.
v8 optimizations #
Jakob Kummerow landed two optimizations to V8 specifically targeted at optimizing React. That's really exciting to see browser vendors helping out on performance!
Reusable Components by Khan Academy #
Khan Academy released many high quality standalone components they are using. This is a good opportunity to see what React code used in production look like.
var TeX = require('react-components/js/tex.jsx');
React.renderComponent(<TeX>\nabla \cdot E = 4 \pi \rho</TeX>, domNode);
var translated = (
<$_ first="Motoko" last="Kusanagi">
Hello, %(first)s %(last)s!
</$_>
);
React + Browserify + Gulp #
Trường wrote a little guide to help your getting started using React, Browserify and Gulp.
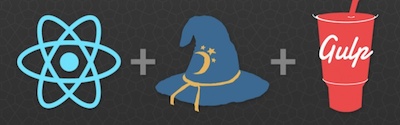
React Style #
After React put HTML inside of JavaScript, Sander Spies takes the same approach with CSS: IntegratedCSS. It seems weird at first but this is the direction where React is heading.
var Button = React.createClass({
normalStyle: ReactStyle(function() {
return { backgroundColor: vars.orange };
}),
activeStyle: ReactStyle(function() {
if (this.state.active) {
return { color: 'yellow', padding: '10px' };
}
}),
render: function() {
return (
<div styles={[this.normalStyle(), this.activeStyle()]}>
Hello, I'm styled
</div>
);
}
});
Virtual DOM in Elm #
Evan Czaplicki explains how Elm implements the idea of a Virtual DOM and a diffing algorithm. This is great to see React ideas spread to other languages.
Performance is a good hook, but the real benefit is that this approach leads to code that is easier to understand and maintain. In short, it becomes very simple to create reusable HTML widgets and abstract out common patterns. This is why people with larger code bases should be interested in virtual DOM approaches.
Components Tutorial #
If you are getting started with React, Joe Maddalone made a good tutorial on how to build your first component.
Saving time & staying sane? #
When Kent William Innholt who works at M>Path summed up his experience using React in an article.
We're building an ambitious new web app, where the UI complexity represents most of the app's complexity overall. It includes a tremendous amount of UI widgets as well as a lot rules on what-to-show-when. This is exactly the sort of situation React.js was built to simplify.
- Big win: Tighter coupling of markup and behavior
- Jury's still out: CSS lives outside React.js
- Big win: Cascading updates and functional thinking
- Jury's still out: Verbose propagation
Weather #
To finish this round-up, Andrew Gleave made a page that displays the Weather. It's great to see that React is also used for small prototypes.
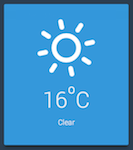